Most of all popular websites on internet use the technique of AJAX, it’s important to clarify that AJAX is NOT a programming language, as the subtitle says it’s a technique.
AJAX technique is helpful when you try to manipulate information without reloading the entire page. As we know AJAX is the acronym of Asynchronous JavaScript And XML so while all the functions are working with Javascript, the access data works with XMLHttpRequest. AJAX is an asynchronous technology in the sense that the additional data is requested to the server and this information loads on the background without “touching” the views and the rest of the page. By this way is possible interact with the information displayed without reloading the site, getting a better speed and usability on the applications.
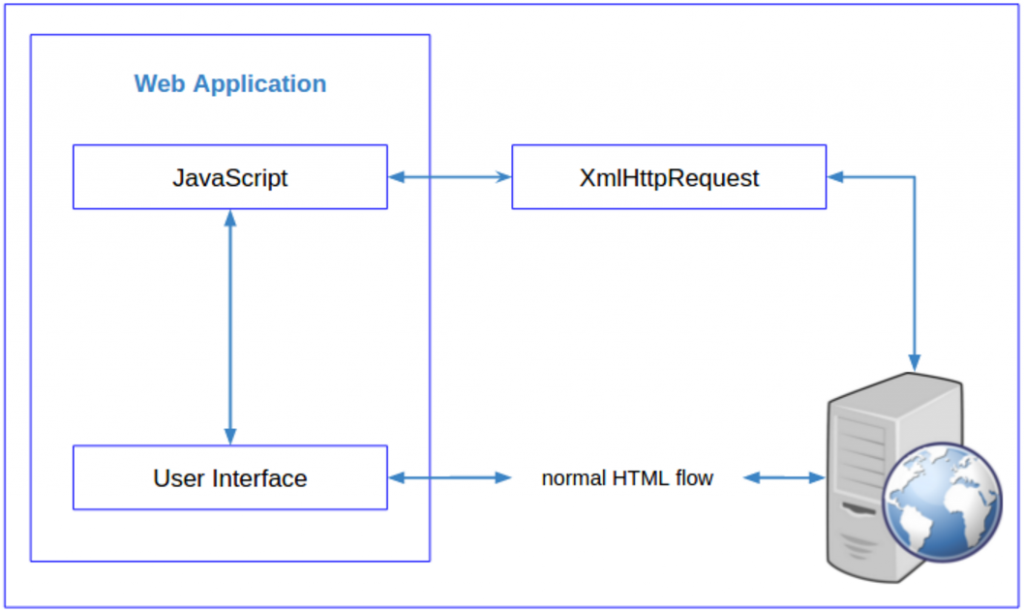
AJAX can work with different languages like php and of course javascript and js libraries commonly with JQuery Library, also AJAX works with techniques like json and can be implemented on different frameworks, as the developer wishes.
How AJAX work?
The following image describes the process request when someone uses AJAX
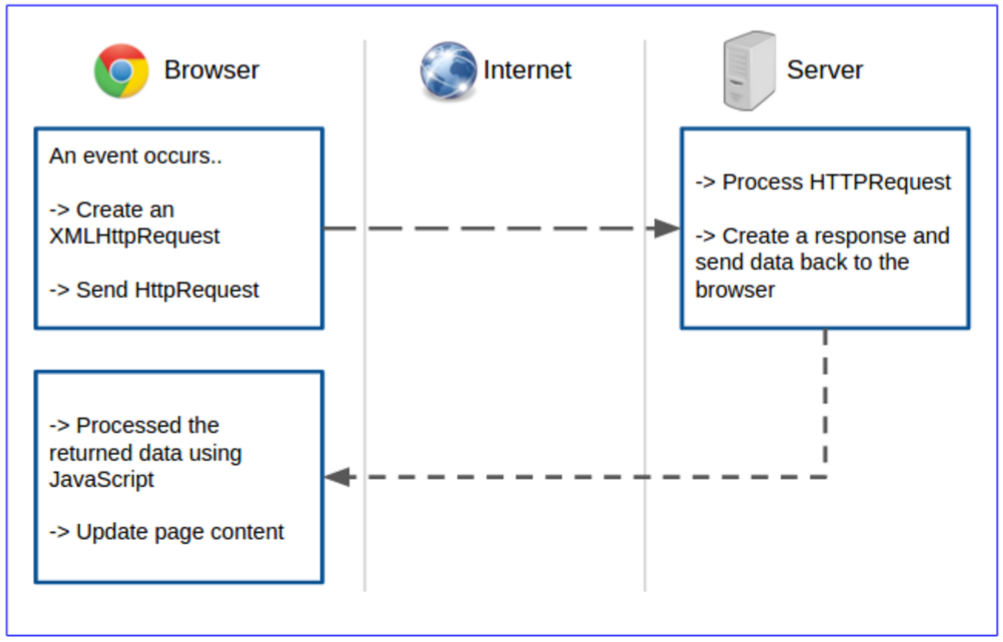
AJAX and JQuery
On JQuery there are many different methods to produce AJAX, those methods can be useful but it depends on what task is going to be solved,and the application’s needs. JQuery documentation divides these methods on “High Level” and “Low Level” .
The Low Level functions are closer to the browser AJAX, more customizable and more powerful. The High Level functions have an interface and are simpler, but also those are less powerful because they were created for a use defined.
In all cases most of JQuery functions are High Level except JQuery.AJAX() which is Low Level along with other methods that usually we are not going to use.
JQuery.AJAX()
This is a method that we can use to make any kind of behavior for our project, also we can find this method as $.AJAX() so JQuery.AJAX() it’s equals to $.AJAX()
The following example uses JQuery AJAX to obtain a json object from the url “http://api.openweathermap.org/data/2.1/weather/city/caracas?type=json“.
The parameters used are:
- url: The address where the info will be sent
- type: Type of request, can be GET, POST , PUT, DELETE. In case of use POST or PUT we can send an object or other parameter to the same function calling “data”, for example data: { ‘key’ : ‘value’ }
- datatype: The type of response expected.
<!DOCTYPE html> <html> <head> <!-- Here we are calling to JQuery library --> <script src="http://code.jquery.com/jquery-2.1.0.min.js"></script> <meta charset="utf-8"> <title>jQuery Ajax</title> <script> function testAjax(){ $.ajax({ //JQuery.AJAX() method //the url wher the data will be sending, in this case is a api url url: 'http: //api.openwheathermap.org/data/2.1/weather/city/caracas', type: 'GET', //se epecify what kind of request are using dataType: "jsonp", //the data type in this case JSON success: function(data){ //if the function success obtain the info $('body').append('' + JSON.stringify(data, null, 2) + ''); }, error: function(jqXHR, testStatus, error){ //in case that error occurs alert("error: " + jqXHR.responseText); } }); } </script> </head> <body> <!-- Our button that are calling the method testAjax() --> <button onclick="testAJAX();">Click to test</button> </body> </html>
So if we test our page and click on the test button the data is displayed
<script type="text/javascript"> $("#element").load("mypage.html"); </script>
.load()
This method can be used to load data on a website element. Just indicates the URL of the file that we want and the method update the HTML of the element. This method doesn’t accept any parameter and just makes the function of load, nothing else.
<script type="text/javascript"> $("#element").load("mypage.html"); </script>
Also we can send data by GET method, just indicates the data inside the url.
<script type="text/javascript"> $("#element").load("mypage.php?data=12qw"); </script>
$.get() and $.post()
Two brother methods that do the same task: an AJAX connection sending data to the server. The difference is that $.get() send data via GET (in the url) and $.post() send data via POST (in HTTP headers).
On these methods we send parameters but it is not an obligation, just the first one.
<script type="text/javascript"> $.get("url.php", { data1: "value1", data2: "value2" }, function(response){ $("#result").html(data); }, "html"); </script>
$.getScript()
This method loads a JavaScript file in a specific address
<script type="text/javascript"> $.getScript("www.myurl.com/ajax/filejs.js", function(data, textStatus, jqxhr){ alert("success"); }); </script>
This is equal to use:
<script type="text/javascript"> $.ajax({ url: "http://myURL.com/ajax/myscript.js", dataType: "script", success: function(data, textStatus, jqxhr){ alert("Success"); } }); </script>
$.getJSON
Here we specify the response type , in case of expect jsonp you must add ‘callback=?’ to the url.
<script type="text/javascript"> $.getJSON("http://api.openweathermap.org/data/2.1/weather/city/caracas?callback=?", function(){ alert("success"); }); </script>
And with $.ajax() method is equal to:
<script type="text/javascript"> $.ajax({ url: "http://api.openweathermap.org/data/2.1/weather/city/caracas", type: 'GET', dataType: "script", success: function(){ alert("Success"); } }); </script>
This is a global vision on how AJAX and JQuery work I hope this post was clear and you can understand the importance of this technique when you want to develop a project. In the next entry blog we can develop an application with ajax and if you have any doubts send us an email or Contact Us Here!”